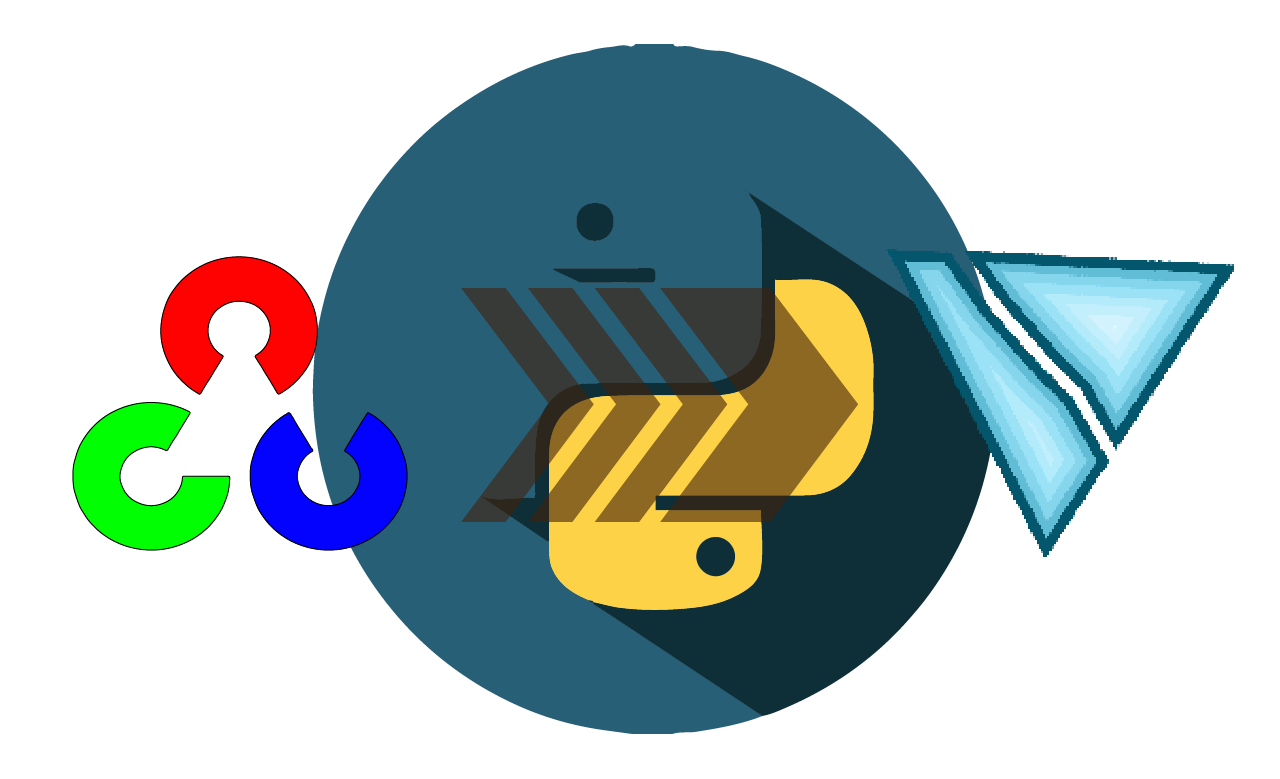
Switching from OpenCV Library¶
Switching OpenCV with VidGear APIs is fairly painless process, and will just require changing a few lines in your python script.
This document is intended to software developers who want to migrate their python code from OpenCV Library to VidGear APIs.
Prior knowledge of Python or OpenCV won't be covered in this guide. Proficiency with OpenCV-Python (Python API for OpenCV) is a must in order understand this document.
If you're just getting started with OpenCV-Python programming, then refer this FAQ âž¶
Why VidGear is better than OpenCV?¶
Learn more about OpenCV here âž¶
VidGear employs OpenCV at its backend and enhances its existing capabilities even further by introducing many new state-of-the-art functionalities such as:
- Accelerated Multi-Threaded Performance.
- Out-of-the-box support for OpenCV APIs.
- Real-time Stabilization ready.
- Lossless hardware enabled video encoding and transcoding.
- Inherited multi-backend support for various video sources and devices.
- Screen-casting, Multi-bitrate network-streaming, and way much more âž¶
Vidgear offers all this at once while maintaining the same standard OpenCV-Python (Python API for OpenCV) coding syntax for all of its APIs, thereby making it even easier to implement complex real-time OpenCV applications in python code without changing things much.
Switching the VideoCapture APIs¶
Let's compare a bare-minimum python code for extracting frames out of any Webcam/USB-camera (connected at index 0), between OpenCV's VideoCapture Class and VidGear's CamGear VideoCapture API side-by-side:
CamGear API share the same syntax as other VideoCapture APIs, thereby you can easily switch to any of those APIs in a similar manner.
# import required libraries
import cv2
# Open suitable video stream, such as webcam on first index(i.e. 0)
stream = cv2.VideoCapture(0)
# loop over
while True:
# read frames from stream
(grabbed, frame) = stream.read()
# check for frame if not grabbed
if not grabbed:
break
# {do something with the frame here}
# Show output window
cv2.imshow("Output", frame)
# check for 'q' key if pressed
key = cv2.waitKey(1) & 0xFF
if key == ord("q"):
break
# close output window
cv2.destroyAllWindows()
# safely close video stream
stream.release()
# import required libraries
from vidgear.gears import CamGear
import cv2
# Open suitable video stream, such as webcam on first index(i.e. 0)
stream = CamGear(source=0).start()
# loop over
while True:
# read frames from stream
frame = stream.read()
# check for frame if Nonetype
if frame is None:
break
# {do something with the frame here}
# Show output window
cv2.imshow("Output", frame)
# check for 'q' key if pressed
key = cv2.waitKey(1) & 0xFF
if key == ord("q"):
break
# close output window
cv2.destroyAllWindows()
# safely close video stream
stream.stop()
and both syntax almost looks the same, easy, isn't it?
Differences¶
Let's breakdown a few noteworthy difference in both syntaxes:
Task | OpenCV VideoCapture Class | VidGear's CamGear API |
---|---|---|
Initiating | stream = cv2.VideoCapture(0) | stream = CamGear(source=0).start() |
Reading frames | (grabbed, frame) = stream.read() | frame = stream.read() |
Checking empty frame | if not grabbed: | if frame is None: |
Terminating | stream.release() | stream.stop() |
Now checkout other VideoCapture Gears âž¶
Switching the VideoWriter API¶
Let's extend previous bare-minimum python code and save those extracted frames to disk as a valid file, with OpenCV's VideoWriter Class and VidGear's WriteGear (with FFmpeg backend), compared side-to-side:
WriteGear API also provides backend for OpenCV's VideoWriter Class. More information here âž¶
# import required libraries
import cv2
# Open suitable video stream, such as webcam on first index(i.e. 0)
stream = cv2.VideoCapture(0)
# Define the codec and create VideoWriter object with suitable output
# filename for e.g. `Output.avi`
fourcc = cv2.VideoWriter_fourcc(*'XVID')
writer = cv2.VideoWriter('output.avi', fourcc, 20.0, (640, 480))
# loop over
while True:
# read frames from stream
(grabbed, frame) = stream.read()
# check for frame if not grabbed
if not grabbed:
break
# {do something with the frame here}
# write frame to writer
writer.write(frame)
# Show output window
cv2.imshow("Output", frame)
# check for 'q' key if pressed
key = cv2.waitKey(1) & 0xFF
if key == ord("q"):
break
# close output window
cv2.destroyAllWindows()
# safely close video stream
stream.release()
# safely close writer
writer.release()
# import required libraries
from vidgear.gears import CamGear
from vidgear.gears import WriteGear
import cv2
# Open suitable video stream, such as webcam on first index(i.e. 0)
stream = CamGear(source=0).start()
# Define WriteGear Object with suitable output filename for e.g. `Output.mp4`
writer = WriteGear(output = 'Output.mp4')
# loop over
while True:
# read frames from stream
frame = stream.read()
# check for frame if None-type
if frame is None:
break
# {do something with the frame here}
# write frame to writer
writer.write(frame)
# Show output window
cv2.imshow("Output Frame", frame)
# check for 'q' key if pressed
key = cv2.waitKey(1) & 0xFF
if key == ord("q"):
break
# close output window
cv2.destroyAllWindows()
# safely close video stream
stream.stop()
# safely close writer
writer.close()
Noticed WriteGear's coding syntax looks similar but less complex?
Differences¶
Let's breakdown a few noteworthy difference in both syntaxes:
Task | OpenCV VideoWriter Class | VidGear's WriteGear API |
---|---|---|
Initiating | writer = cv2.VideoWriter('output.avi', cv2.VideoWriter_fourcc(*'XVID'), 20.0, (640, 480)) | writer = WriteGear(output='Output.mp4') |
Writing frames | writer.write(frame) | writer.write(frame) |
Terminating | writer.release() | writer.close() |
Now checkout more about WriteGear API here âž¶